80+ Most Asked Javascript Interview Questions(TCS, Accenture, IBM, Infosys, etc)
3 min readSep 22, 2022
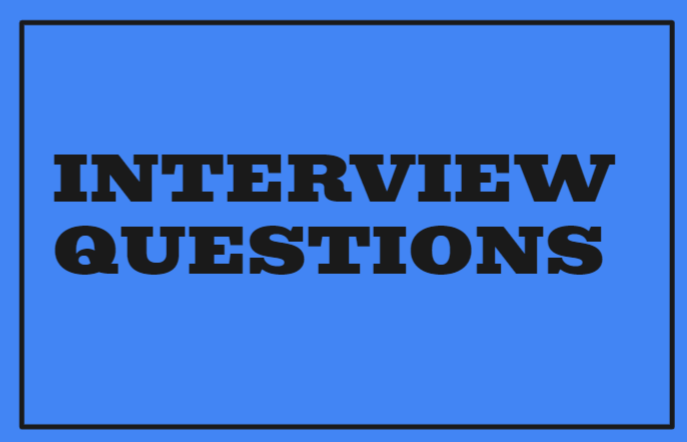
- What is event bubbling in JavaScript?
- Explain bind, call and apply.
- Difference between map and for each.
- What is hoisting?
- Can you share the code of call and bind?
- What is the difference between bind and call?
- What is this keyword?
- Explain Callback & Callback hell
- The difference between callback, promise, and async-await
- Can you explain async-await and its advantages?
- Explain Clouser in javaScript.
- Explain curry in javaScript.
- Explain memory leak in JavaScript.
- Internal working mechanism for JavaScript.
- About the project and its scalability.
- What is the lexical scope?
- Explain the different methods of promise & also explain when to use them. (promise.all, promise.allSettled etc)
- Different ways to create objects in JavaScript. (there are 4 ways )
- Explained the design pattern & how u have implemented it in your project also?
- What is a higher-order function?
- What is the event emitter, and how do emit and listen to events?
- What is a prototype in JavaScript?
- What will u get a prototype of the object?
- Explain cors?
- What is the return value of reduce?
- Is javaScript have a map data type?
- Are you using OOPS in JavaScript?
- What is Functional programming?
- Explain the difference between for loop & array.map, when to use them.
- Explain the javaScript engine execution flow by taking below example: setTimeout(console.log()) and setImmeditae(console.log());
- Describe JavaScript execution stack and event loop functionality.
- How you can invoke HTTP calls from the server itself (superset modules)?
- how you are handling dependency injections?
- Can you pls explain the lifecycles of HTTP?
- What is the middleware and where you have used it in your project? any example?
- What is a callback, and why do we need it?
- What are the modules you are using all over the project?
- Explain a few methods of arrays.
- What are destructuring and spread Operators and their benefits of using it?
- What is better to write in code, callback, or promises? Why?
- How you are doing testing in your team?
- Why did you choose apache JMeter over other testing tools?
- How we can use inheritance in JavaScript?
- Why do we need JavaScript?
- Difference between require and import.
- What is some and every in JavaScript?
- What is event bubbling and capturing?
- What are obj.freeze and obj.seal & difference between them?
- What are the best ways to create objects?
- Benefits of creating an object using Object.create
- What is the state for a promise?
- Which latest ECMA standard replaces promise?
- How to handle catch in async await?
- How to handle catch in async await without using try-catch?
- Which tool you are using for coding & why?
- How do you debug the code?
- How u learn new things & new stack?
- Which modules you are using for creating HTTP services & why?
- What is the difference between undefined and null?
- What are interpolations in JavaScript?
- Why nodeJs was created as a single-threaded language?
- What are shallow cloning and deep cloning & the difference between them?
- What is the second value in parseInt?
- What are event emitters and dispatchers?
- What is the answer to a given question why? [] == [];
- Difference between const vs Object.freeze() vs Object.seal()
- How you are invoking APIs from the front end?
- What is the best way to copy the object and why?
- How to deep clone?
- Inbuilt methods of cloning the object vs loadash_clone? which one is better & why?
- What is the optimized way to sort the array?
- Explain the different types of sorting Algorithms.
- Is In-built JS sorting methods better or user custom sorting? explain why also?
- What is the best way to create an object in the given option Object.create vs creating an object from new.
- Adding a field to the const object is possible.
- Can values be added to the const array?
- How have singleton design patterns been implemented (at the code level)?
- What distinguishes the arrow function from the standard function?
- How do spread operators work?
- Describe the ES6 feature.
- What are some benefits of traditional for loop constructs vs for each?
- What is the difference between this in an arrow function and a traditional function?
- What are some dangers of closures?